Android permissions aim to protect the privacy of an Android user. They prompt the user when apps want to access data types for sensitive data like contacts or photos, and system features like location or step detection. Users grant these permissions when first downloading your app.
If your app needs access to any of these data types (a subset of the Google Fit data types), request the relevant Android permission before requesting the OAuth permissions. See below.
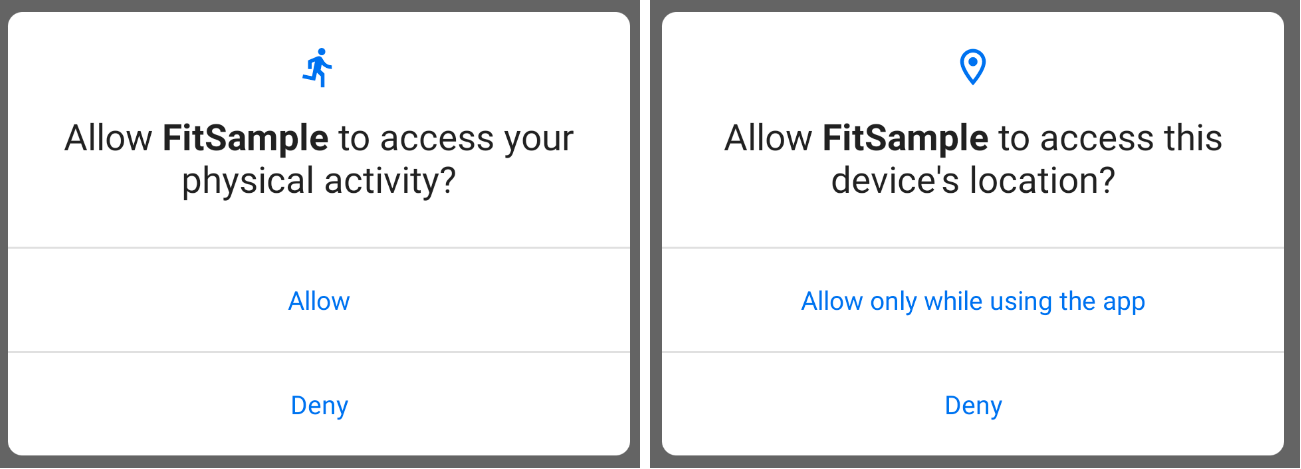
Data types that need Android permissions
To access these physical activity data types, you'll need to request the ACTIVITY_RECOGNITION
Android permission:
To record these data types:
com.google.step_count.delta
com.google.step_count.cumulative
com.google.step_count.cadence
com.google.activity.segment
com.google.calories.expended
To read these data types:
com.google.step_count.delta
com.google.step_count.cumulative
com.google.step_count.cadence
com.google.activity.segment
com.google.activity.exercise
To access these data types, you'll need to request the ACCESS_FINE_LOCATION
Android permission:
- To read these data types:
com.google.distance.delta
com.google.location.sample
com.google.location.bounding_box
com.google.speed
To record these data types, you'll need to request the BODY_SENSORS
Android permission:
com.google.heart_rate.bpm
Requesting Android permissions
Learn about requesting Android permissions, the physical activity recognition permission, the fine location permission, and the body sensors permission.
To access the data types above with the Google Fit APIs, you'll need to
implement logic to handle requesting Android permissions for both Android 10 and
previous versions of Android. These examples use the ACTIVITY_RECOGNITION
permission.
Android 10
So your app can target API level 29 or above, request the permission from the user, and register the permission in the application manifest file.
Add the permission to the manifest file.
<uses-permission android:name="android.permission.ACTIVITY_RECOGNITION"/>
Check if the permission is granted:
if (ContextCompat.checkSelfPermission(thisActivity, Manifest.permission.ACTIVITY_RECOGNITION) != PackageManager.PERMISSION_GRANTED) { // Permission is not granted }
If permission isn't already granted, request the permission:
ActivityCompat.requestPermissions(thisActivity, arrayOf(Manifest.permission.ACTIVITY_RECOGNITION), MY_PERMISSIONS_REQUEST_ACTIVITY_RECOGNITION)
Android 9 and below
So your app can target API level 28 or below:
Request the
com.google.android.gms.permission.ACTIVITY_RECOGNITION
permission.Add the permission to the manifest file.
<uses-permission android:name="android.gms.permission.ACTIVITY_RECOGNITION"/>