このガイドでは、Google Chat API の Message
リソースで create()
メソッドを使用して、次のいずれかを行う方法について説明します。
- テキスト、カード、インタラクティブなウィジェットを含むメッセージを送信します。
- 特定の Chat ユーザーに非公開でメッセージを送信する。
- メッセージ スレッドを開始または返信する。
- 他の Chat API リクエストで指定できるように、メッセージに名前を付けます。
メッセージの最大サイズ(テキストやカードを含む)は 32,000 バイトです。このサイズを超えるメッセージを送信するには、Chat アプリが複数のメッセージを送信する必要があります。
Chat アプリは、Chat API を呼び出してメッセージを作成するだけでなく、ユーザーの操作に返信するメッセージを作成して送信することもできます。たとえば、ユーザーが Chat アプリをスペースに追加した後にウェルカム メッセージを投稿できます。インタラクションに応答する際に、Chat アプリは、インタラクティブなダイアログやリンク プレビュー インターフェースなど、他のタイプのメッセージ機能を使用できます。ユーザーに返信するには、Chat アプリは Chat API を呼び出すことなく、メッセージを同期的に返します。インタラクションに応答するメッセージを送信する方法については、Google Chat アプリでインタラクションを受け取って応答するをご覧ください。
Chat API で作成されたメッセージを Chat がどのように表示し、属性付けするか
create()
メソッドは、アプリ認証とユーザー認証を使用して呼び出すことができます。Chat では、使用する認証の種類に応じてメッセージの送信者を異なります。
Chat アプリとして認証すると、Chat アプリがメッセージを送信します。
App
が表示されます。ユーザーとして認証すると、Chat アプリがユーザーに代わってメッセージを送信します。また、Chat アプリの名前を表示して、メッセージに Chat アプリを関連付けます。
認証タイプによって、メッセージに含めることができるメッセージ機能とインターフェースも決まります。アプリ認証を使用すると、Chat アプリはリッチテキスト、カードベースのインターフェース、インタラクティブなウィジェットを含むメッセージを送信できます。Chat ユーザーはメッセージにテキストのみを送信できるため、ユーザー認証を使用してメッセージを作成する場合にのみテキストを含めることができます。Chat API で利用可能なメッセージ機能の詳細については、Google Chat メッセージの概要をご覧ください。
このガイドでは、どちらの認証タイプを使用して Chat API でメッセージを送信するかについて説明します。
前提条件
Node.js
- Google Chat へのアクセス権を持つ Business または Enterprise の Google Workspace アカウント。
- 環境を設定します。
- Google Cloud プロジェクトを作成します。
- OAuth 同意画面を構成する
- Google Chat API を有効にして構成し、Chat アプリの名前、アイコン、説明を指定します。
- Node.js の Cloud クライアント ライブラリをインストールします。
- Google Chat API リクエストで認証する方法に基づいてアクセス認証情報を作成します。
- Chat ユーザーとして認証するには、OAuth クライアント ID 認証情報を作成し、認証情報を
credentials.json
という名前の JSON ファイルとしてローカル ディレクトリに保存します。 - Chat アプリとして認証するには、サービス アカウントの認証情報を作成し、認証情報を
credentials.json
という名前の JSON ファイルとして保存します。
- Chat ユーザーとして認証するには、OAuth クライアント ID 認証情報を作成し、認証情報を
- ユーザーとして認証するか、Chat アプリとして認証するかに応じて、 認可スコープを選択します。
- 認証済みユーザーまたは呼び出し元の Chat アプリがメンバーである Google Chat スペース。Chat アプリとして認証するには、Chat アプリをスペースに追加します。
Python
- Google Chat へのアクセス権を持つ Business または Enterprise の Google Workspace アカウント。
- 環境を設定します。
- Google Cloud プロジェクトを作成します。
- OAuth 同意画面を構成する
- Google Chat API を有効にして構成し、Chat アプリの名前、アイコン、説明を指定します。
- Python の Cloud クライアント ライブラリをインストールします。
- Google Chat API リクエストで認証する方法に基づいてアクセス認証情報を作成します。
- Chat ユーザーとして認証するには、OAuth クライアント ID 認証情報を作成し、認証情報を
credentials.json
という名前の JSON ファイルとしてローカル ディレクトリに保存します。 - Chat アプリとして認証するには、サービス アカウントの認証情報を作成し、認証情報を
credentials.json
という名前の JSON ファイルとして保存します。
- Chat ユーザーとして認証するには、OAuth クライアント ID 認証情報を作成し、認証情報を
- ユーザーとして認証するか、Chat アプリとして認証するかに応じて、 認可スコープを選択します。
- 認証済みユーザーまたは呼び出し元の Chat アプリがメンバーである Google Chat スペース。Chat アプリとして認証するには、Chat アプリをスペースに追加します。
Java
- Google Chat へのアクセス権を持つ Business または Enterprise の Google Workspace アカウント。
- 環境を設定します。
- Google Cloud プロジェクトを作成します。
- OAuth 同意画面を構成する
- Google Chat API を有効にして構成し、Chat アプリの名前、アイコン、説明を指定します。
- Java の Cloud クライアント ライブラリをインストールします。
- Google Chat API リクエストで認証する方法に基づいてアクセス認証情報を作成します。
- Chat ユーザーとして認証するには、OAuth クライアント ID 認証情報を作成し、認証情報を
credentials.json
という名前の JSON ファイルとしてローカル ディレクトリに保存します。 - Chat アプリとして認証するには、サービス アカウントの認証情報を作成し、認証情報を
credentials.json
という名前の JSON ファイルとして保存します。
- Chat ユーザーとして認証するには、OAuth クライアント ID 認証情報を作成し、認証情報を
- ユーザーとして認証するか、Chat アプリとして認証するかに応じて、 認可スコープを選択します。
- 認証済みユーザーまたは呼び出し元の Chat アプリがメンバーである Google Chat スペース。Chat アプリとして認証するには、Chat アプリをスペースに追加します。
Apps Script
- Google Chat へのアクセス権を持つ Business または Enterprise の Google Workspace アカウント。
- 環境を設定します。
- Google Cloud プロジェクトを作成します。
- OAuth 同意画面を構成する
- Google Chat API を有効にして構成し、Chat アプリの名前、アイコン、説明を指定します。
- スタンドアロンの Apps Script プロジェクトを作成し、Advanced Chat Service を有効にします。
- このガイドでは、ユーザー認証またはアプリ認証のいずれかを使用する必要があります。Chat アプリとして認証するには、サービス アカウントの認証情報を作成します。手順については、Google Chat アプリとして認証と認可を行うをご覧ください。
- ユーザーとして認証するか、Chat アプリとして認証するかに応じて、 認可スコープを選択します。
- 認証済みユーザーまたは呼び出し元の Chat アプリがメンバーである Google Chat スペース。Chat アプリとして認証するには、Chat アプリをスペースに追加します。
Chat アプリとしてメッセージを送信する
このセクションでは、アプリ認証を使用して、テキスト、カード、インタラクティブなアクセサリ ウィジェットを含むメッセージを送信する方法について説明します。
アプリ認証を使用して CreateMessage()
メソッドを呼び出すには、リクエストで次のフィールドを指定する必要があります。
chat.bot
認可スコープ。- メッセージを投稿する
Space
リソース。Chat アプリがスペースのメンバーである必要があります。 - 作成する
Message
リソース。メッセージの内容を定義するには、リッチテキスト(text
)、1 つ以上のカード インターフェース(cardsV2
)、またはその両方を追加します。
必要に応じて、次を含めることができます。
accessoryWidgets
フィールド: メッセージの下部にインタラクティブなボタンを追加します。privateMessageViewer
フィールド: 指定したユーザーにメッセージを非公開で送信します。messageId
フィールド。他の API リクエストで使用するメッセージに名前を付けることができます。thread.threadKey
フィールドとmessageReplyOption
フィールド: スレッドを開始または返信します。スペースでスレッド処理を使用していない場合は、このフィールドは無視されます。
次のコードは、Chat アプリとして投稿されたメッセージ(テキスト、カード、メッセージの下部にあるクリック可能なボタンを含む)を Chat アプリが送信する方法の例を示しています。
Node.js
Python
Java
Apps Script
このサンプルを実行するには、SPACE_NAME
をスペースの name
フィールドの ID に置き換えます。ID は、ListSpaces()
メソッドを呼び出すか、スペースの URL から取得できます。
メッセージの下部にインタラクティブなウィジェットを追加する
このガイドの最初のコードサンプルでは、Chat アプリのメッセージの下部に、アクセサリ ウィジェットと呼ばれるクリック可能なボタンが表示されます。アクセサリ ウィジェットは、メッセージ内のテキストまたはカードの後に表示されます。これらのウィジェットを使用すると、次のようなさまざまな方法でユーザーにメッセージを操作するよう促すことができます。
- メッセージの正確性や満足度を評価する。
- メッセージ アプリまたは Chat アプリに関する問題を報告する。
- ドキュメントなどの関連コンテンツへのリンクを開く。
- チャットアプリから類似のメッセージを特定の期間閉じるか、スヌーズする。
アクセサリ ウィジェットを追加するには、リクエストの本文に accessoryWidgets[]
フィールドを含め、追加するウィジェットを 1 つ以上指定します。
次の画像は、アクセサリ ウィジェットをテキスト メッセージに追加して、ユーザーが Chat アプリの使用感を評価できるようにした Chat アプリを示しています。
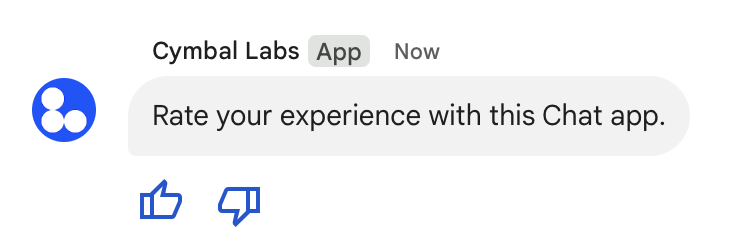
次の例は、2 つのアクセサリ ボタンを含むテキスト メッセージを作成するリクエストの本文を示しています。ユーザーがボタンをクリックすると、対応する関数(doUpvote
など)が操作を処理します。
{
text: "Rate your experience with this Chat app.",
accessoryWidgets: [{ buttonList: { buttons: [{
icon: { material_icon: {
name: "thumb_up"
}},
color: { red: 0, blue: 255, green: 0 },
onClick: { action: {
function: "doUpvote"
}}
}, {
icon: { material_icon: {
name: "thumb_down"
}},
color: { red: 0, blue: 255, green: 0 },
onClick: { action: {
function: "doDownvote"
}}
}]}}]
}
メッセージを非公開で送信する
チャットアプリでは、メッセージを非公開で送信して、スペース内の特定のユーザーにのみ表示することができます。チャットアプリが非公開メッセージを送信すると、そのメッセージには、そのメッセージが自分だけに表示されることを伝えるラベルが表示されます。
Chat API を使用して非公開でメッセージを送信するには、リクエストの本文に privateMessageViewer
フィールドを指定します。ユーザーを指定するには、値を Chat ユーザーを表す User
リソースに設定します。次の例に示すように、User
リソースの name
フィールドを使用することもできます。
{
text: "Hello private world!",
privateMessageViewer: {
name: "users/USER_ID"
}
}
このサンプルを使用するには、USER_ID
をユーザーの一意の ID(12345678987654321
や hao@cymbalgroup.com
など)に置き換えます。ユーザーの指定の詳細については、Google Chat ユーザーを特定して指定するをご覧ください。
メッセージを非公開で送信するには、リクエストで次の項目を省略する必要があります。
ユーザーに代わってテキストメッセージを送信する
このセクションでは、ユーザー認証を使用してユーザーに代わってメッセージを送信する方法について説明します。ユーザー認証では、メッセージの内容に含めることができるのはテキストのみで、カード インターフェースやインタラクティブなウィジェットなど、Chat アプリでのみ使用できるメッセージ機能は省略する必要があります。
ユーザー認証を使用して CreateMessage()
メソッドを呼び出すには、リクエストで次のフィールドを指定する必要があります。
- このメソッドのユーザー認証をサポートする認可スコープ。次のサンプルでは、
chat.messages.create
スコープを使用します。 - メッセージを投稿する
Space
リソース。認証されたユーザーは、スペースのメンバーである必要があります。 - 作成する
Message
リソース。メッセージの内容を定義するには、text
フィールドを含める必要があります。
必要に応じて、次を含めることができます。
messageId
フィールド。他の API リクエストで使用するメッセージに名前を付けることができます。thread.threadKey
フィールドとmessageReplyOption
フィールド: スレッドを開始または返信します。スペースでスレッド処理を使用していない場合は、このフィールドは無視されます。
次のコードは、Chat アプリが認証済みユーザーに代わって特定のスペースでテキスト メッセージを送信する方法の例を示しています。
Node.js
Python
Java
Apps Script
このサンプルを実行するには、SPACE_NAME
をスペースの name
フィールドの ID に置き換えます。ID は、ListSpaces()
メソッドを呼び出すか、スペースの URL から取得できます。
スレッドを開始する、またはスレッドに返信する
スレッドを使用するスペースでは、新しいメッセージがスレッドを開始するか、既存のスレッドに返信するかを指定できます。
デフォルトでは、Chat API を使用して作成したメッセージは新しいスレッドを開始します。スレッドを特定して後で返信できるように、リクエストでスレッドキーを指定できます。
- リクエストの本文で
thread.threadKey
フィールドを指定します。 - クエリ パラメータ
messageReplyOption
を指定して、キーがすでに存在する場合の動作を決定します。
既存のスレッドに返信するメッセージを作成するには:
- リクエストの本文に、
thread
フィールドを含めます。設定する場合は、作成したthreadKey
を指定できます。それ以外の場合は、スレッドのname
を使用する必要があります。 - クエリ パラメータ
messageReplyOption
を指定します。
次のコードは、Chat アプリが認証済みユーザーに代わって、特定のスペースのキーで識別される特定のスレッドを開始または返信するテキスト メッセージを送信する方法の例を示しています。
Node.js
Python
Java
Apps Script
このサンプルを実行するには、次のように置き換えます。
THREAD_KEY
: スペース内の既存のスレッドキー。または、新しいスレッドを作成する場合は、スレッドの一意の名前。SPACE_NAME
: スペースのname
フィールドの ID。ID は、ListSpaces()
メソッドを呼び出すか、スペースの URL から取得できます。
メッセージに名前を付ける
今後の API 呼び出しでメッセージを取得または指定するには、リクエストで messageId
フィールドを設定してメッセージに名前を付けます。メッセージに名前を付けると、メッセージのリソース名(name
フィールドで表されます)からシステム割り当て ID を保存しなくても、メッセージを指定できます。
たとえば、get()
メソッドを使用してメッセージを取得するには、リソース名を使用して取得するメッセージを指定します。リソース名の形式は spaces/{space}/messages/{message}
です。ここで、{message}
は、システム割り当て ID またはメッセージの作成時に設定したカスタム名を表します。
メッセージに名前を付けるには、メッセージを作成するときに messageId
フィールドにカスタム ID を指定します。messageId
フィールドには、Message
リソースの clientAssignedMessageId
フィールドの値を設定します。
メッセージに名前を付けられるのは、メッセージの作成時のみです。既存のメッセージのカスタム ID に名前を付けたり、カスタム ID を変更したりすることはできません。カスタム ID は次の要件を満たしている必要があります。
client-
で始まります。たとえば、client-custom-name
は有効なカスタム ID ですが、custom-name
は有効ではありません。- 63 文字以下で、小文字、数字、ハイフンのみを使用できます。
- スペース内で一意です。チャットアプリでは、異なるメッセージに同じカスタム ID を使用できません。
次のコードは、Chat アプリが認証済みユーザーに代わって、ID を含むテキスト メッセージを特定のスペースに送信する方法の例を示しています。
Node.js
Python
Java
Apps Script
このサンプルを実行するには、次のように置き換えます。
SPACE_NAME
: スペースのname
フィールドの ID。ID は、ListSpaces()
メソッドを呼び出すか、スペースの URL から取得できます。MESSAGE-ID
:custom-
で始まるメッセージの名前。指定したスペースで Chat アプリによって作成された他のメッセージ名と重複しない必要があります。
トラブルシューティング
Google Chat アプリまたはカードからエラーが返されると、Chat インターフェースに「エラーが発生しました」というメッセージが表示されます。または「リクエストを処理できません」というメッセージが表示されます。Chat UI にエラー メッセージが表示されない場合でも、Chat アプリまたはカードで予期しない結果が生成されることがあります(カード メッセージが表示されないなど)。
エラー メッセージが Chat UI に表示されない場合がありますが、Chat アプリのエラー ロギングがオンになっている場合は、説明的なエラー メッセージとログデータを使用してエラーを修正できます。エラーの表示、デバッグ、修正については、Google Chat エラーのトラブルシューティングと修正をご覧ください。
関連トピック
- カードビルダーを使用すると、Chat アプリ用の JSON カード メッセージを設計してプレビューできます。
- メッセージの書式を設定する。
- メッセージの詳細を取得する。
- スペース内のメッセージを一覧表示する。
- メッセージを更新する。
- メッセージを削除する。
- Google Chat のメッセージでユーザーを特定する。
- 着信 Webhook を使用して Google Chat にメッセージを送信する。