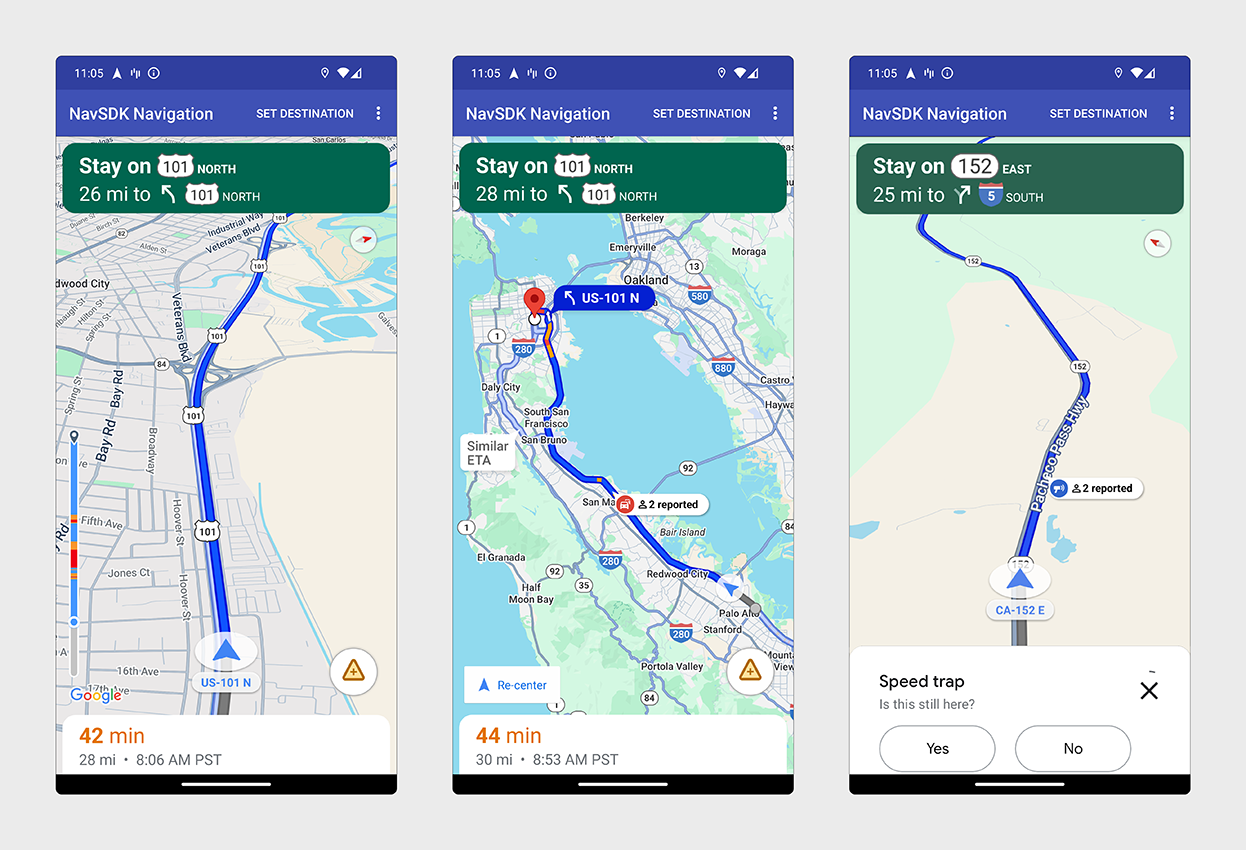
Navigation SDK for Android
Build dynamic navigation experiences for your Android apps.
Get Started
Start building with commonly used features of the Navigation SDK for Android.
Set up your project
Create an account, generate an API key, and start building.
Navigate your first route
Learn how to use the Navigation SDK to navigate a single-destination route within your app.
Modify the navigation UI
Customize which UI elements and controls appear during the navigation experience in your app.
Listen for navigation events
Listen and respond to navigation events that occur as users navigate along a route using your app.
Features
Learn about core features of the Navigation SDK for Android.
Google Navigation Experience
Add the Google navigation experience to your app.
Custom Navigation Experience
Leverage the Google navigation routing to build custom experiences for your app.
Route Experience
Customize route preferences, manage waypoints, and plan routes.
Code Samples and Libraries
Try sample apps that demonstrate the use of the Navigation SDK for Android and explore cross-platform navigation libraries.
Try the demo apps
Run the Navigation SDK demo apps for both the navigation and maps features.
Explore cross-platform libraries
Use libraries for Flutter and React Native to add navigation experiences to your cross-platform apps.