Web Bluetooth API 允许网站与蓝牙设备通信。
如果我告诉你们,网站能够以安全且可保护隐私的方式与附近的蓝牙设备通信,情况会怎样?这样,心率监测器、鸣叫的灯泡,甚至乌龟都可以直接与网站互动。
到目前为止,只有平台专用应用能够与蓝牙设备交互。Web Bluetooth API 旨在改变这种状况,并将其引入网络浏览器。
开始前须知
本文档假定您对蓝牙低功耗 (BLE) 和通用属性配置文件的工作原理有基本的了解。
虽然 Web Bluetooth API 规范尚未最终确定,但规范作者正在积极寻找热心的开发者试用此 API,并提供有关规范的反馈和有关实现的反馈。
Web Bluetooth API 的一部分适用于 ChromeOS、Chrome(Android 版 6.0)、Mac (Chrome 56) 和 Windows 10 (Chrome 70)。这意味着,您应该能够请求和连接附近的蓝牙低功耗设备、读取/写入蓝牙特性、接收 GATT 通知,了解蓝牙设备何时断开连接,甚至对蓝牙描述符执行读写操作。如需了解详情,请参阅 MDN 的浏览器兼容性表格。
对于 Linux 及更早版本的 Windows,请在 about://flags
中启用 #experimental-web-platform-features
标志。
可用于源试用
为了从实际使用 Web Bluetooth API 的开发者那里获得尽可能多的反馈,Chrome 之前已在 Chrome 53 中添加了此功能,作为 ChromeOS、Android 和 Mac 的源试用。
试用期已于 2017 年 1 月成功结束。
安全性要求
如需了解安全方面的权衡因素,建议您阅读 Jeffrey Yasskin(Chrome 团队软件工程师)撰写的网络蓝牙安全模型一文,该博文负责 Web Bluetooth API 规范。
仅限 HTTPS
由于此实验性 API 是添加到 Web 的一项强大新功能,因此它仅供安全上下文使用。这意味着您在构建时需要考虑 TLS。
需要用户手势
作为一项安全功能,具有 navigator.bluetooth.requestDevice
发现的蓝牙设备必须通过用户手势(例如触摸或点击鼠标)触发。我们讨论的是监听 pointerup
、click
和 touchend
事件。
button.addEventListener('pointerup', function(event) {
// Call navigator.bluetooth.requestDevice
});
了解代码
Web Bluetooth API 非常依赖 JavaScript Promise。如果您不熟悉它们,可以参阅这篇精彩的 Promise 教程。此外,() => {}
是 ECMAScript 2015 箭头函数。
请求蓝牙设备
此版本的 Web Bluetooth API 规范允许以中心角色运行的网站通过 BLE 连接连接到远程 GATT 服务器。它支持实现蓝牙 4.0 或更高版本的设备之间的通信。
当某个网站使用 navigator.bluetooth.requestDevice
请求访问附近的设备时,浏览器会通过设备选择器提示用户,用户可以从中选择一台设备或取消该请求。
navigator.bluetooth.requestDevice()
函数接受一个用于定义过滤条件的必需对象。这些过滤器用于仅返回与某些通告的蓝牙 GATT 服务和/或设备名称匹配的设备。
服务过滤条件
例如,如需请求通告蓝牙 GATT 电池服务的蓝牙设备:
navigator.bluetooth.requestDevice({ filters: [{ services: ['battery_service'] }] })
.then(device => { /* … */ })
.catch(error => { console.error(error); });
不过,如果您的蓝牙 GATT 服务不在标准化蓝牙 GATT 服务列表中,您可以提供完整的蓝牙 UUID,或提供较短的 16 位或 32 位格式。
navigator.bluetooth.requestDevice({
filters: [{
services: [0x1234, 0x12345678, '99999999-0000-1000-8000-00805f9b34fb']
}]
})
.then(device => { /* … */ })
.catch(error => { console.error(error); });
名称过滤条件
您还可以根据使用 name
过滤器键通告的设备名称,甚至是具有 namePrefix
过滤器键的此名称的前缀来请求蓝牙设备。请注意,在这种情况下,您还需要定义 optionalServices
键,以便能够访问未包含在服务过滤器中的任何服务。否则,您稍后在尝试访问它们时会收到错误消息。
navigator.bluetooth.requestDevice({
filters: [{
name: 'Francois robot'
}],
optionalServices: ['battery_service'] // Required to access service later.
})
.then(device => { /* … */ })
.catch(error => { console.error(error); });
制造商数据过滤器
此外,还可以根据使用 manufacturerData
过滤器键通告的制造商特定数据请求蓝牙设备。此密钥是一组对象,其中包含一个名为 companyIdentifier
的强制性蓝牙公司标识符密钥。您还可以提供一个数据前缀,用于从以该数据开头的蓝牙设备中过滤制造商数据。请注意,您还需要定义 optionalServices
键,才能访问服务过滤器中未包含的任何服务。否则,您稍后在尝试访问它们时会收到错误消息。
// Filter Bluetooth devices from Google company with manufacturer data bytes
// that start with [0x01, 0x02].
navigator.bluetooth.requestDevice({
filters: [{
manufacturerData: [{
companyIdentifier: 0x00e0,
dataPrefix: new Uint8Array([0x01, 0x02])
}]
}],
optionalServices: ['battery_service'] // Required to access service later.
})
.then(device => { /* … */ })
.catch(error => { console.error(error); });
掩码也可以与数据前缀一起使用,以匹配制造商数据中的某些模式。如需了解详情,请查看蓝牙数据过滤器说明。
排除过滤条件
借助 navigator.bluetooth.requestDevice()
中的 exclusionFilters
选项,您可以从浏览器选择器中排除某些设备。它可用于排除与更广泛的过滤条件匹配但不受支持的设备。
// Request access to a bluetooth device whose name starts with "Created by".
// The device named "Created by Francois" has been reported as unsupported.
navigator.bluetooth.requestDevice({
filters: [{
namePrefix: "Created by"
}],
exclusionFilters: [{
name: "Created by Francois"
}],
optionalServices: ['battery_service'] // Required to access service later.
})
.then(device => { /* … */ })
.catch(error => { console.error(error); });
不使用滤镜
最后,您可以使用 acceptAllDevices
键显示所有附近的蓝牙设备,而不是使用 filters
。您还需要定义 optionalServices
键才能访问某些服务。否则,您稍后在尝试访问它们时会收到错误消息。
navigator.bluetooth.requestDevice({
acceptAllDevices: true,
optionalServices: ['battery_service'] // Required to access service later.
})
.then(device => { /* … */ })
.catch(error => { console.error(error); });
连接到蓝牙设备
有了 BluetoothDevice
,您现在要怎么做?让我们连接到保存服务和特性定义的蓝牙远程 GATT 服务器。
navigator.bluetooth.requestDevice({ filters: [{ services: ['battery_service'] }] })
.then(device => {
// Human-readable name of the device.
console.log(device.name);
// Attempts to connect to remote GATT Server.
return device.gatt.connect();
})
.then(server => { /* … */ })
.catch(error => { console.error(error); });
读取蓝牙特征
在这里,我们连接到远程蓝牙设备的 GATT 服务器。现在,我们想要获取主 GATT 服务并读取属于此服务的特征。例如,我们来尝试读取设备电池的当前电量。
在前面的示例中,battery_level
是标准化的电池电量特性。
navigator.bluetooth.requestDevice({ filters: [{ services: ['battery_service'] }] })
.then(device => device.gatt.connect())
.then(server => {
// Getting Battery Service…
return server.getPrimaryService('battery_service');
})
.then(service => {
// Getting Battery Level Characteristic…
return service.getCharacteristic('battery_level');
})
.then(characteristic => {
// Reading Battery Level…
return characteristic.readValue();
})
.then(value => {
console.log(`Battery percentage is ${value.getUint8(0)}`);
})
.catch(error => { console.error(error); });
如果您使用自定义蓝牙 GATT 特征,则可以向 service.getCharacteristic
提供完整的蓝牙 UUID 或简短的 16 位或 32 位格式。
请注意,您还可以针对某个特征添加 characteristicvaluechanged
事件监听器,以处理其值的读取操作。请参阅读取特征值更改示例,了解如何选择性地处理即将发布的 GATT 通知。
…
.then(characteristic => {
// Set up event listener for when characteristic value changes.
characteristic.addEventListener('characteristicvaluechanged',
handleBatteryLevelChanged);
// Reading Battery Level…
return characteristic.readValue();
})
.catch(error => { console.error(error); });
function handleBatteryLevelChanged(event) {
const batteryLevel = event.target.value.getUint8(0);
console.log('Battery percentage is ' + batteryLevel);
}
写入蓝牙特征
写入蓝牙 GATT 特征与读取一样容易。这一次,我们使用心率控制点将心率监测器设备上“Energy Expended”(能量消耗)字段的值重置为 0。
我保证这里没有魔法。所有这一切都在心率控制点特征页面中给出解释。
navigator.bluetooth.requestDevice({ filters: [{ services: ['heart_rate'] }] })
.then(device => device.gatt.connect())
.then(server => server.getPrimaryService('heart_rate'))
.then(service => service.getCharacteristic('heart_rate_control_point'))
.then(characteristic => {
// Writing 1 is the signal to reset energy expended.
const resetEnergyExpended = Uint8Array.of(1);
return characteristic.writeValue(resetEnergyExpended);
})
.then(_ => {
console.log('Energy expended has been reset.');
})
.catch(error => { console.error(error); });
接收 GATT 通知
现在,我们来看看当设备上的心率测量特性发生变化时如何收到通知:
navigator.bluetooth.requestDevice({ filters: [{ services: ['heart_rate'] }] })
.then(device => device.gatt.connect())
.then(server => server.getPrimaryService('heart_rate'))
.then(service => service.getCharacteristic('heart_rate_measurement'))
.then(characteristic => characteristic.startNotifications())
.then(characteristic => {
characteristic.addEventListener('characteristicvaluechanged',
handleCharacteristicValueChanged);
console.log('Notifications have been started.');
})
.catch(error => { console.error(error); });
function handleCharacteristicValueChanged(event) {
const value = event.target.value;
console.log('Received ' + value);
// TODO: Parse Heart Rate Measurement value.
// See https://github.com/WebBluetoothCG/demos/blob/gh-pages/heart-rate-sensor/heartRateSensor.js
}
通知示例介绍了如何使用 stopNotifications()
停止通知,以及如何正确移除添加的 characteristicvaluechanged
事件监听器。
断开与蓝牙设备的连接
为了提供更好的用户体验,您可能需要监听断开连接事件并邀请用户重新连接:
navigator.bluetooth.requestDevice({ filters: [{ name: 'Francois robot' }] })
.then(device => {
// Set up event listener for when device gets disconnected.
device.addEventListener('gattserverdisconnected', onDisconnected);
// Attempts to connect to remote GATT Server.
return device.gatt.connect();
})
.then(server => { /* … */ })
.catch(error => { console.error(error); });
function onDisconnected(event) {
const device = event.target;
console.log(`Device ${device.name} is disconnected.`);
}
您也可以调用 device.gatt.disconnect()
,断开您的 Web 应用与蓝牙设备的连接。这将触发现有的 gattserverdisconnected
事件监听器。请注意,如果其他应用已在与蓝牙设备通信,则不会停止蓝牙设备通信。如需深入了解,请查看设备断开连接示例和自动重新连接示例。
读取和写入蓝牙描述符
蓝牙 GATT 描述符是描述特征值的属性。您可以采用与蓝牙 GATT 特性类似的方式读取和写入这些信号。
例如,我们来看看如何解读用户对于设备的健康温度计测量间隔的说明。
在以下示例中,health_thermometer
是健康温度计服务,measurement_interval
是测量间隔特征,gatt.characteristic_user_description
是特征用户说明描述符。
navigator.bluetooth.requestDevice({ filters: [{ services: ['health_thermometer'] }] })
.then(device => device.gatt.connect())
.then(server => server.getPrimaryService('health_thermometer'))
.then(service => service.getCharacteristic('measurement_interval'))
.then(characteristic => characteristic.getDescriptor('gatt.characteristic_user_description'))
.then(descriptor => descriptor.readValue())
.then(value => {
const decoder = new TextDecoder('utf-8');
console.log(`User Description: ${decoder.decode(value)}`);
})
.catch(error => { console.error(error); });
现在,我们已经阅读了用户对设备健康温度计测量间隔的说明,接下来我们了解一下如何对其进行更新并写入自定义值。
navigator.bluetooth.requestDevice({ filters: [{ services: ['health_thermometer'] }] })
.then(device => device.gatt.connect())
.then(server => server.getPrimaryService('health_thermometer'))
.then(service => service.getCharacteristic('measurement_interval'))
.then(characteristic => characteristic.getDescriptor('gatt.characteristic_user_description'))
.then(descriptor => {
const encoder = new TextEncoder('utf-8');
const userDescription = encoder.encode('Defines the time between measurements.');
return descriptor.writeValue(userDescription);
})
.catch(error => { console.error(error); });
示例、演示和 Codelab
以下所有网络蓝牙示例均已成功完成测试。为了充分利用这些示例,建议您安装 [BLE 外设模拟器 Android 应用],该应用可通过电池服务、心率服务或健康温度计服务模拟 BLE 外围设备。
新手
- 设备信息 - 从 BLE 设备检索基本设备信息。
- 电池电量 - 从发布电池信息的 BLE 设备中检索电池信息。
- 重置能量 - 重置 BLE 设备通告心率消耗的能量。
- 特征属性 - 显示 BLE 设备中特定特征的所有属性。
- 通知 - 开始和停止来自 BLE 设备的特征通知。
- 设备断开连接 - 断开 BLE 设备,并在连接至 BLE 设备后断开连接时会收到通知。
- 获取特征 - 从 BLE 设备获取所通告服务的所有特征。
- 获取描述符 - 从 BLE 设备获取所通告服务的所有特征描述符。
- 制造商数据过滤器 - 从 BLE 设备中检索与制造商数据匹配的基本设备信息。
- 排除过滤器 - 从具有基本排除过滤器的 BLE 设备中检索基本设备信息。
组合多项操作
- GAP 特性 - 获取 BLE 设备的所有 GAP 特性。
- 设备信息特征 - 获取 BLE 设备的所有设备信息特征。
- 链路损失 - 设置 BLE 设备的警报级别特征(readValue 和 writeValue)。
- 发现服务和特征 - 发现 BLE 设备中所有可访问的主要服务及其特征。
- 自动重新连接 - 使用指数退避算法重新连接到断开连接的 BLE 设备。
- 读取特征值已更改 - 读取电池电量,并在 BLE 设备发生更改时收到通知。
- 读取描述符 - 从 BLE 设备读取服务的所有特征的描述符。
- 写入描述符 - 写入 BLE 设备上的“特征用户描述”描述符。
您也可以查看我们精选的 Web 蓝牙演示和官方的 Web 蓝牙 Codelab。
库
- web-bluetooth-utils 是一个 npm 模块,用于向 API 添加一些便捷函数。
- noble 提供了 Web Bluetooth API shim,它是最热门的 Node.js BLE 中央模块。这样,您无需 WebSocket 服务器或其他插件即可进行 webpack/browserify 开发。
- Angular-web-bluetooth 是一个用于 Angular 的模块,它抽象了配置 Web Bluetooth API 所需的所有样板。
工具
- Web Bluetooth 使用入门是一款简单的 Web 应用,它将生成所有 JavaScript 样板代码以开始与蓝牙设备交互。输入设备名称、服务和特征,定义其属性,然后就可以了。
- 如果您已经是蓝牙开发者,Web Bluetooth Developer Studio 插件也会为您的蓝牙设备生成网络蓝牙 JavaScript 代码。
提示
Chrome 中的 about://bluetooth-internals
提供了 Bluetooth Internals 页面,以便您检查与附近蓝牙设备相关的所有内容:状态、服务、特征和描述符。
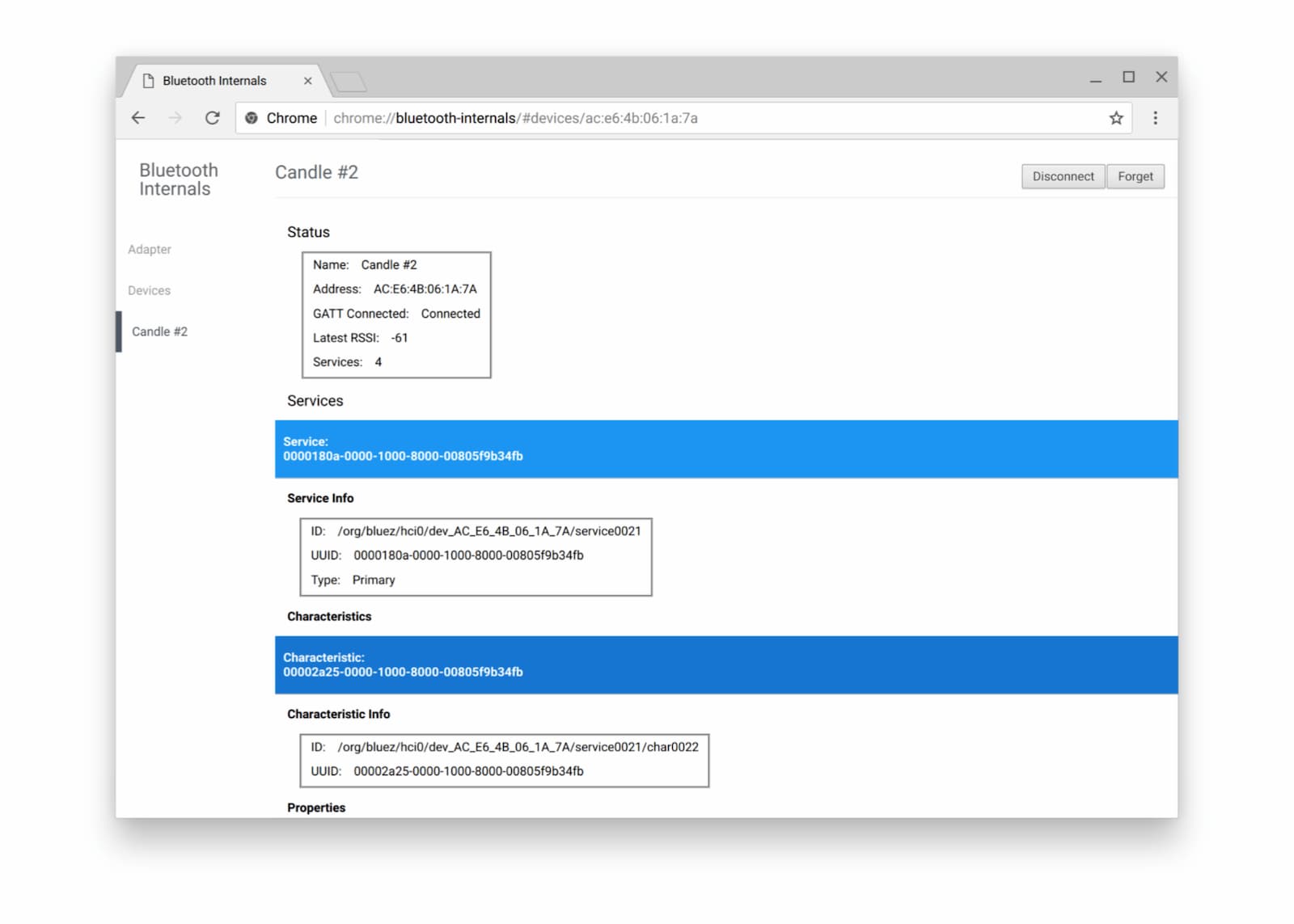
我还建议您查看官方的如何提交 Web 蓝牙 bug 页面,因为调试蓝牙有时可能很困难。
后续步骤
请先查看浏览器和平台实现状态,以了解 Web Bluetooth API 的哪些部分目前正在实现中。
虽然尚未完成,但下面是对近期未来发展的先睹为快:
- 扫描附近的 BLE 通告将通过
navigator.bluetooth.requestLEScan()
执行。 - 新的
serviceadded
事件将跟踪新发现的蓝牙 GATT 服务,而serviceremoved
事件将跟踪已移除的蓝牙 GATT 服务。在蓝牙 GATT 服务中添加或移除任何特征和/或描述符时,会触发新的servicechanged
事件。
显示对该 API 的支持
您打算使用 Web Bluetooth API 吗?您的公开支持有助于 Chrome 团队确定功能的优先级,并向其他浏览器供应商显示支持这些功能的重要性。
请使用 # 标签 #WebBluetooth
向 @ChromiumDev 发送一条推文,并告诉我们您使用该产品的位置和方式。
资源
致谢
感谢 Kayce Basques 审核本文。 主打图片由美国博尔德市的 SparkFun 电子产品提供。